go 小白一枚,建了一个关于go的微信交流群
欢迎大家加入,加我微信拉群
微信号:XU1014129578
暗号 :gogogo
### 方法的定义
```
func (recevier type) methodName(参数列表) (返回值列表){
方法体
return 返回值
}
```
### 方法的使用
1. 方法需要个定义的类型进行绑定
```
type Person struct {
Name string
}
func (p Person)testMothod(){ //绑定P类型
fmt.Println("Name =",p.Name)
}
func main() {
person := Person{Name:"jack"}
person.testMothod()
}
```
1. golang中的方法是作用在指定的数据类型上的(即:和指定的数据类型绑定),因此自定义类型,都可以有方法,而不仅仅是struct
例如:`type number int`
number 也是可以绑定方法的
1. 方法只能通过定义的类型的变量来调用,而不能直接调用,也不能使用其他类型变量来调用
```
type Person struct {
Name string
}
type Car struct {
Price int
}
func (p Person)testMothod(){
fmt.Println("Name =",p.Name)
}
func main() {
//正确调用
person := Person{Name:"jack"}
person.testMothod()
//错误调用
car := Car{Price:5000000}
car.testMothod() //只能使用Person类型调用,使用Car调用就会报错
}
```
### 方法和函数的区别
1. 调用方式不一样
函数调用方式:函数名(实参列表)
方法调用方式: 变量.方法名(实参列表)
代码:
```
package main
import (
"fmt"
)
type Person struct {
Name string
}
//函数
func testFunc(p Person){
fmt.Println("函数中的Name = ",p.Name)
}
//方法
func (p Person) testMethod(){
fmt.Println("方法中的Name = ",p.Name)
}
func main(){
//函数调用方式
person := Person{Name:"Jack"}
testFunc(person)
//方法调用方式
person.Name = "Pon"
person.testMethod()
}
```
运行结果:
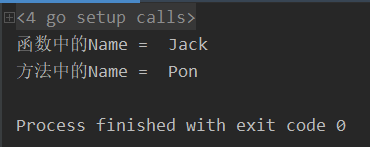
2.对于普通函数,接收则为值类型时,不能将指针类型的数据直接传递,反之亦然
```
type Person struct {
Name string
}
func testFunc01(p Person){
fmt.Println("函数中的Name = ",p.Name)
}
func testFunc02(p *Person){
fmt.Println("函数中的Name = ",p.Name)
}
func main(){
//函数调用
person := Person{Name:"Jack"}
testFunc01(person)
testFunc02(&person) //这里如果testFunc02(person) 这样调用就会报错
}
```
3.对于方法(如struct的方法),接收者为值类型时,可以直接用指针类型的变量调用方法,反过来同样也可以
```
type Person struct {
Name string
}
func (p Person) testMethod01(){
fmt.Println("方法中的Name = ",p.Name)
}
func (p *Person) testMethod02(){
fmt.Println("方法中的Name = ",p.Name)
}
func main(){
//方法调用
person := Person{Name:"Jack"}
person.testMethod01() //这里 person.testMethod01() 和 (&person).testMethod01() 调用都是一样的,都是值拷贝
(&person).testMethod02() //这里 person.testMethod02() 和 (&person).testMethod02() 调用都是一样的,都是地址拷贝
}
```
总结:
不管调用形式如何,真正决定是值拷贝还是地址拷贝,看这个方法和那个类型绑定。
如果是和值类型,比如(p Person),则是值拷贝,如果和指针类型,比如(p *Person)则是地址拷贝
有疑问加站长微信联系(非本文作者))
