改版了下书上的计算目录下所有总目录的文件大小,执行后小目录的可以跑出结果,但是根盘就不行,报错,求大佬指导
```
import (
"fmt"
"os"
"path/filepath"
"sync"
"time"
)
var done = make(chan struct{})
func cancelled() bool {
select {
case <-done:
return true
default:
return false
}
}
func main() {
// Determine the initial directories.
roots := os.Args[1:]
if len(roots) == 0 {
roots = []string{"."}
}
go func() {
os.Stdin.Read(make([]byte, 1)) // read a single byte
close(done)
}()
fileSizes := make(chan map[string]int64)
var n sync.WaitGroup
for _, root := range roots {
n.Add(1)
go walkDir(root, &n, fileSizes)
}
go func() {
n.Wait()
close(fileSizes)
}()
tick := time.Tick(500 * time.Millisecond)
var nbytes = make(map[string]int64)
loop:
for {
select {
case <-done:
// Drain fileSizes to allow existing goroutines to finish.
for range fileSizes {
// Do nothing.
}
return
case size, ok := <-fileSizes:
if !ok {
break loop // fileSizes was closed
}
for k, v := range size {
nbytes[k] += v
}
case <-tick:
//printDiskUsage(nfiles, nbytes)
}
}
for k, v := range nbytes {
if v > 10*1e9 {
printDiskUsage(k, v) // final totals
}
}
}
func printDiskUsage(nfiles string, nbytes int64) {
fmt.Printf("【%s】:【%.1f GB】\n", nfiles, float64(nbytes)/1e9)
}
func walkDir(dir string, n *sync.WaitGroup, fileSizes chan<- map[string]int64) {
dirsSize := make(map[string]int64) //需要有个缓冲,不然并发报错
defer n.Done()
if cancelled() {
return
}
for _, entry := range dirents(dir) {
if entry.IsDir() {
n.Add(1)
subdir := filepath.Join(dir, entry.Name())
go walkDir(subdir, n, fileSizes)
} else {
dirsSize[dir] += entry.Size()
}
fileSizes <- dirsSize
}
}
var sema = make(chan struct{}, 20) // concurrency-limiting counting semaphore
func dirents(dir string) []os.FileInfo {
select {
case sema <- struct{}{}: // acquire token
case <-done:
return nil // cancelled
}
defer func() { <-sema }() // release token
f, err := os.Open(dir)
if err != nil {
fmt.Fprintf(os.Stderr, "du: %v\n", err)
return nil
}
defer f.Close()
entries, err := f.Readdir(0) // 0 => no limit; read all entries
if err != nil {
fmt.Fprintf(os.Stderr, "du: %v\n", err)
// Don't return: Readdir may return partial results.
}
return entries
}
```
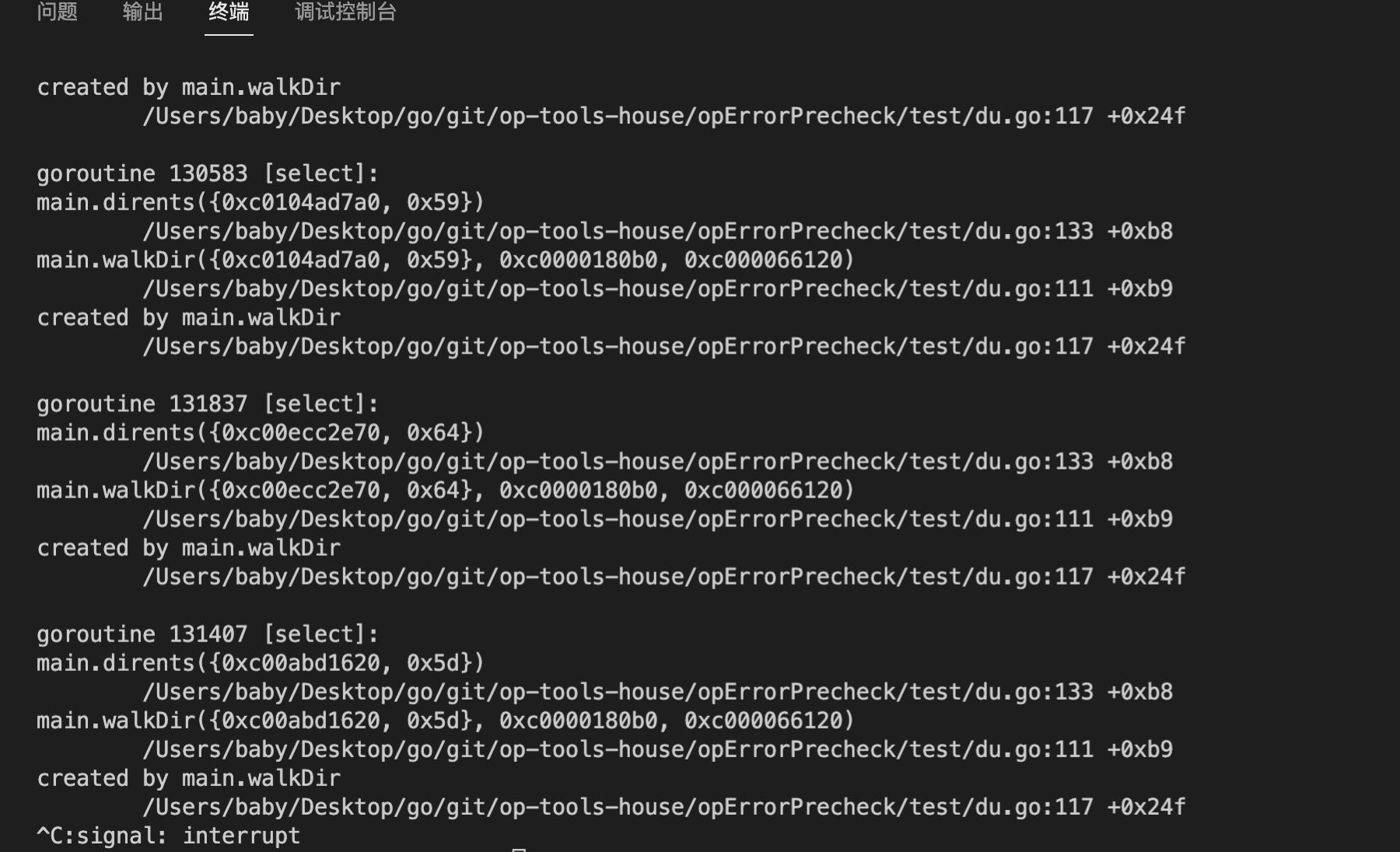
如果我猜得不错,你 go routine 溢出了
如果我猜的不错,你这是 Windows 系统
如果我猜得不错,你处理自己测试的目录没有问题,你全局处理就出错了
如果我猜得不错,你没有处理 dir 类型的 Symlinks 文件
#1
更多评论
1楼 <a href="/user/Mericusta" title="@Mericusta">@Mericusta</a> 为啥会溢出呢?是并发没控制好吗?这电脑是 mac 的,全局文件就有问题,symlinks是啥?软连接?
#2
溢出是因为,针对这种目录类“符号链接”文件,你访问它可能会导致递归,因为它可能指向某一个你的父级目录节点
mac 系统,应该也有对应的文件类型,你可以去了解了解 mac 的文件文件系统应该跟 unix/linux 差不多,找找这种“符号链接”或叫做“软/硬链接”类的文件该如何识别
#3